Prerequisites for learning React JS
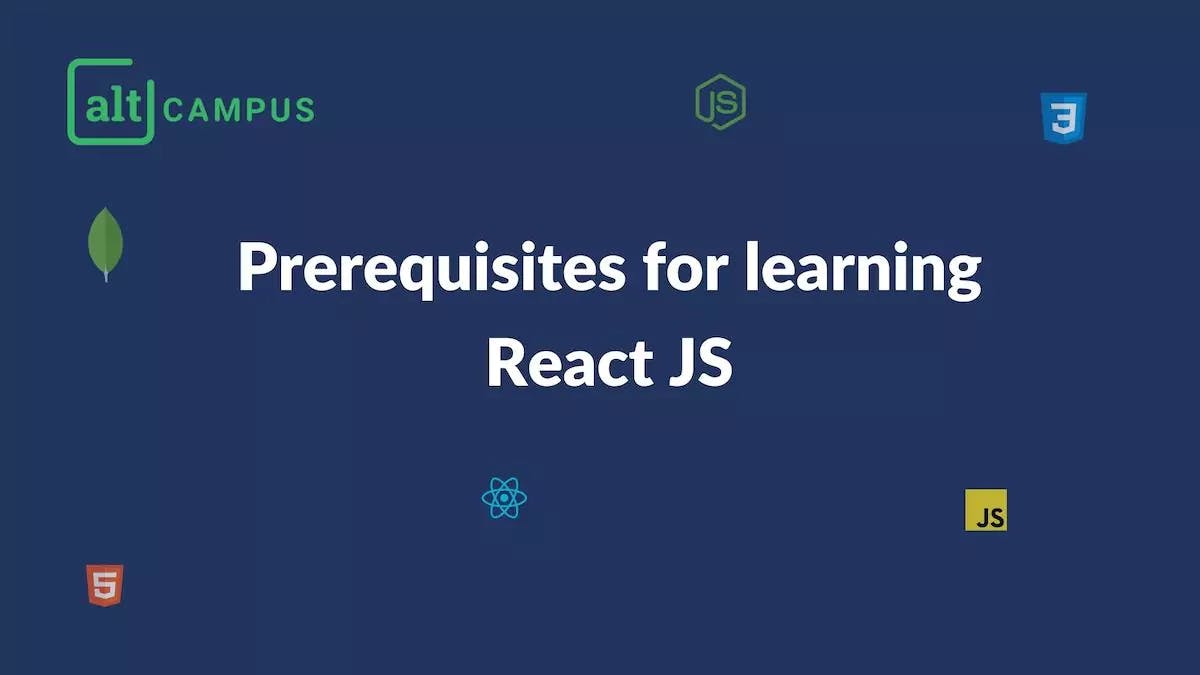
ReactJS is a JavaScript library for making user interfaces. While learning, you will be using other web technologies like HTML, CSS, and JavaScript. So, to learn about ReactJS properly you should be familiar with the following technologies:
- HTML and CSS
- Fundamentals of Javascript, ES6 and beyond
- Node and NPM
Now, that we know the different technologies that we need before learning ReactJS let's look at these in a bit more detail.
HTML
These are the following concepts that you need to know in HTML:
- Different HTML element for headings, lists, images, links, forms etc
- Nesting elements
- HTML Semantic Elements like header, nav, footer, section etc
- Element attributes like class, id, src, href etc
- Building structure using multiple HTML elements
CSS
These are the following concepts that you need to know in CSS:
- Different types of selectors like type, class, id etc
- Grouping selectors
- Cascading nature of CSS
- Calculating Specificity
- Common CSS properties for color, length, background etc
- Box Model
- Positioning
- Flexbox
- Typography
- Handling Media, Lists, Tables, Forms
JavaScript
Being a better ReactJS engineer is fully dependent on how well you understand different JavaScript concepts. The better you are with JavaScript, the better you will understand and use React. These are the following JavaScript concepts you should master before learning ReactJS:
- Values and different types
- Functions as first-class citizens in JavaScript
- Operation on data structures like array and object
- Array, String, and Function methods
- Callback and Higher order functions
- Scope and Closure
- Basics of DOM
- Prototypal nature of objects
- Understanding 'this' keyword
- Prototypal inheritance
- ES6 Class
- 'this' keyword and DOM
- Event Loop
- Promises
- Async/Await
- Declarative vs Imperative programming
- Spread and Rest operators
- ES Modules
- Node package manager
Node and NPM
While you will be working with ReactJS you will be using a lot of external packages created by the community for different use-cases. For this you need to understand the following things about how Node and NPM works:
- What is Node?
- How does NPM work?
- Initializing a folder with NPM
- Installing external packages using NPM
If you are someone new to all of this, take a look at our course which takes you from absolute beginner to becoming a full-stack developer and getting your first developer job. It includes everything (assignments, video lectures, quizzes, interview questions and job prepration) you will need for your journey of becoming a full-stack developer.